Start Learning Python
Start Learning Python
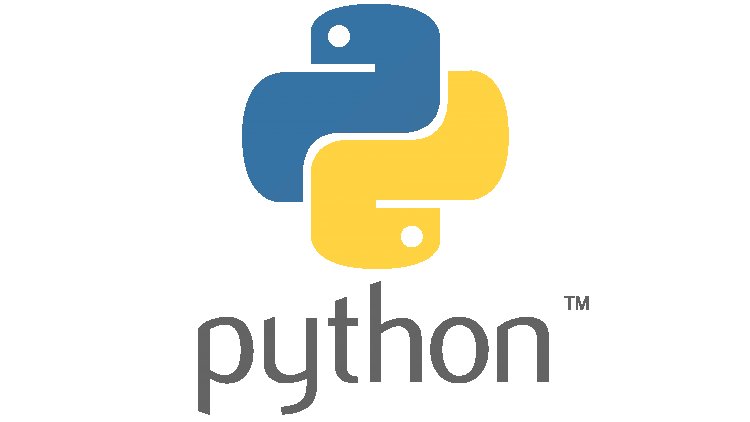
Python is a popular programming language known for its simplicity and readability. It is widely used for web development, data analysis, artificial intelligence, and more.
To get started, I'll provide you with a brief introduction and cover some fundamental concepts. Let's dive in!
1. **Installing Python**: First, you need to install Python on your computer. Visit the official Python website (python.org) and download the latest version compatible with your operating system. Follow the installation instructions to set it up.
2. **Python Interpreter**: Python programs are executed using a Python interpreter. You can interact with Python directly through a command-line interface or use an integrated development environment (IDE) like PyCharm or Visual Studio Code.
3. **Basic Syntax**: Python uses indentation to define blocks of code, so proper indentation is crucial. Statements that belong to the same block should have the same indentation level.
4. **Variables and Data Types**: In Python, you don't need to explicitly declare variables or specify their data types. You can assign values to variables directly. Here's an example:
```python
# Assigning values to variables
message = "Hello, World!"
count = 10
pi = 3.14
```
Python supports various data types, including strings, integers, floats, booleans, lists, dictionaries, and more. You can perform operations and manipulate these data types.
5. **Control Flow**: Python provides control flow statements to control the flow of execution in a program. Common control flow structures include if-else statements, loops, and functions.
```python
# Example of an if-else statement
num = 10
if num > 0:
print("Positive number")
elif num == 0:
print("Zero")
else:
print("Negative number")
```
6. **Functions**: Functions are reusable blocks of code that perform a specific task. They allow you to break your code into smaller, manageable pieces. Here's an example:
```python
# Function definition
def greet(name):
print("Hello, " + name + "!")
# Function call
greet("Alice")
```
7. **Lists**: A list is an ordered collection of items. It can store different types of elements and can be modified. You can access and manipulate list items using indices. Here's an example:
```python
# Creating a list
fruits = ["apple", "banana", "cherry"]
# Accessing list items
print(fruits[0]) # Output: apple
# Modifying list items
fruits[1] = "grape"
print(fruits) # Output: ["apple", "grape", "cherry"]
```
These are just a few fundamental concepts to get you started with Python. As you progress, you can explore more advanced topics like object-oriented programming, file handling, modules, and libraries.
To enhance your learning experience, I recommend practicing coding exercises and working on small projects. There are many online resources, tutorials, and interactive coding platforms available to help you learn Python effectively.
Feel free to ask specific questions or let me know what topics you'd like to explore further. I'm here to assist you on your Python learning journey!